ASCII animation
ASCII Animation is a method in which symbols are manipulated to appear as moving shapes. ASCII drawings are drawn manually with the keyboard on a text editor like Notepad, and it's based on many frames that have the same number of lines(height). Each frame represents one tiny movement of the subject that is animated.
After creating the frames, it has to start showing them sequentially with a specific FPS (Frames Per Second) using a tool such as GIF, a programming language (Python,C/C++, ...), or any other tool, and if you want to learn more about the animation process, check out the ASCII tutorial of "Martian Rex" in this link : stonestoryrpg.com/ascii_tutorial.html
Simple example
we're going to use "Python" to create this animation using this function :
import os
from time import sleep
def animation(frams:str):
FPS = 0.1 # the speed of frames
frams = frams.split("split")
while True:
# looping through all the frames
for fr in frams :
os.system("clear")
print(fr)
sleep(FPS)
_____ |***| |***| ======= (o.o) |=| ___|___ (: //.=|=.\\ // // .=|=. \\// // .=|=. \/ (@ (_=_) | |! !| | || || | () () | || || | || || " ==' '==
then we're going to draw the frames in a multi-line string in a variable called "mrSkeleton" (you call it whatever you want it's not necessarily) and put the word "split" at the end of each frame, then give it to the animation function.
Remember : each frame must have the same height(lines).
# Notice each frame has 18 lines, in this example...
mrSkeleton = r"""
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. //
(@ (_=_)//
| |! !:)
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. //
(@ (_=_)//
| |! !:)
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(-.-)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. ||
(@ (_=_) ||
| |! !| ;:
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. \\
(@ (_=_) \\
| |! !| (:
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. ===(:
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___ (:
//.=|=.\\ //
// .=|=. \\//
// .=|=. \/
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
(●.●) \\
|=| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
.-. ||
(●.●) ||
|-| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
.-. //
GOOD (●.●) //
EVENING! |=| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
.-. //
GOOD (●.●) //
EVENING! |-| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
.-. //
GOOD (●.●) //
EVENING! |=| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
.-. //
(●.●) //
|=| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
.-. ||
(●.●) ||
|=| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======:)
(-.-) \\
|=| //
___|___//
//.=|=. /
// .=|=.
// .=|=.
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___ (:
//.=|=.\\ //
// .=|=. \\//
// .=|=. \/
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. ===(:
(@ (_=_)
| |! !|
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. \\
(@ (_=_) \\
| |! !| (:
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. ||
(@ (_=_) ||
| |! !| ;:
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. //
(@ (_=_)//
| |! !:)
| || ||
| () ()
| || ||
| || ||
" ==' '==
split
_____
|***|
|***|
=======
(●.●)
|=|
___|___
//.=|=.\\
// .=|=. \\
// .=|=. //
(@ (_=_)//
| |! !:)
| || ||
| () ()
| || ||
| || ||
" ==' '==
"""
animation(mrSkeleton) # calling the function
Here is another example in baseball that has the same idea :
/ / <<0 o _O \ /\ /\ /| / / | \ jgs^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^"
C programming animation
Donut math
One of the most popular codes in C in ASCII art is the donut code in 2006 by Andy Sloan who used Maths and linear algebra to code this program and made it look like a donut, here is it :
k;double sin()
,cos();main(){float A=
0,B=0,i,j,z[1760];char b[
1760];printf("\x1b[2J");for(;;
){memset(b,32,1760);memset(z,0,7040)
;for(j=0;6.28>j;j+=0.07)for(i=0;6.28
>i;i+=0.02){float c=sin(i),d=cos(j),e=
sin(A),f=sin(j),g=cos(A),h=d+2,D=1/(c*
h*e+f*g+5),l=cos (i),m=cos(B),n=s\
in(B),t=c*h*g-f* e;int x=40+30*D*
(l*h*m-t*n),y= 12+15*D*(l*h*n
+t*m),o=x+80*y, N=8*((f*e-c*d*g
)*m-c*d*e-f*g-l *d*n);if(22>y&&
y>0&&x>0&&80>x&&D>z[o]){z[o]=D;;;b[o]=
".,-~:;=!*#$@"[N>0?N:0];}}/*#****!!-*/
printf("\x1b[H");for(k=0;1761>k;k++)
putchar(k%80?b[k]:10);A+=0.04;B+=
0.02;}}/*****####*******!!=;:~
~::==!!!**********!!!==::-
.,~~;;;========;;;:~-.
..,--------,*/
$@@@@@$$$$ $$@@@$$$$$###**** #$$$$$$$$$###**!!===! #$$$$$$$$####*!!=;;::;; !###$$$$$####**!==;:~~~~:: *##########***!!=;:--,.,-:: !*#########****!==;~-....,~: =!***######****!!=;:~......-:: =!************!!==;:-.....,~:: =!!**********!!!=;;:-...,-~:;;: ;=!!********!!!==;;:~,-~;====!; ;==!!!!!!!!!!!!===;:~;*##$$##*; ;==!!!!!!!!!!!!===;::-#$@@@$#*; ~;===!!!!!!!!!====;;:~*#$$$$#*; :;;===!=!!!=====;;;::::!*##*!~ ~:;;;===========;;;;:::;=!!!;- ~::;;;=======;;;;;;:::;;==;~ -~::;;;;;;;;;;;;;;::::::::- --~::::;;;;;::::::::::::- -~~~~::::::::::~~~~~-, ,---~~~~~~~~~-~-,,
if you want to know how it works, he has an article explaining this code :
a1k0n.net/2011/07/20/donut-math.htmlASCII solid rotation
This is a code from IOCCC in 2013 by Yusuke Endoh, and it's a 3D convex polyhedron viewer which reads three-dimensional vertices from stdin, calculates a convex hull of them, and renders it using ASCII characters "',;;;,;'".
There is a "solids.tbz2" file that includes various solid data in (.txt) files you can render using endoh4.c file, and you can find it in their link : ioccc.org/years-spoiler.html#2013_endoh4
how to use endoh4 :
1. compile endoh4.c with this command , and you can also change the size in the command :
gcc -DS=120,40 -o endoh4 endoh4.cyou must have gcc compiler for C, and be sure solids and endoh4.c are in the same folder.
2. then render a text file with ./endoh4, here is an example:
cat solids/catalan-solid/c10-triakis-icosahedron.txt | ./endoh4ASCII Fluid
Endoh has another program called “Fluid” in 2012 and it is a fluid simulator using the “Smoothed-particle hydrodynamics (SPH)” method, this obfuscated program deals with complex numbers and produces animated ASCII graphics.
there are many text files included in Fluid that you can render with endoh1.c file in this link : ioccc.org/years.html#2012_endoh1
how to execut it :
compile endoh1.c with this command, like the previous one :
gcc endoh1.c -DG=1 -DP=4 -DV=4 -D_BSD_SOURCE -o endoh1 -lmthen render it with ./endoh1 using the cat command, or use a one of the text files like clock.txt.
cat endoh1.c | ./endoh1,_____.,_______.,____.,__.,___. ,___.,___., |/\/\#||#######||####\/\#||/\#| ,.,. |\/\||/\/'| |||||#||#######||#####||#||||#| ,__/||\__. ,/#||||||| | |\/||/\/###\###||#####\/#||||#| |\/\||/\#| ||\||||||| | |##||||#\####\#||##/-\\#\||||#| |#||||||#| |||||||||\.| |##|||||\|\|---'`--' |#|\||||#\. |#\/||\/#| |||||||||#|| |##\/||||||| ,/\|||||||\| `--\||/-'' ,/\/\/||||#|| |#\##||\/\/\_____. |||||||||\/|,.,. `'`' ,_/\#|\#||\/#|| |#/\#\/#\########\__/\/||||||#\\/||\__. ,___.,__.,__.,___. ,__/##\#\/#\/#/'\/ `-\/\/\################||||\/####||/\#| ||\#||##||##||#/\|,__//\#########|\\.|# ,_/\#\/##\#######\#\###||||#|\###||||#| |||#\/\#||##\/#||\/\##\//\\\\##\#\/#||# |#|||\####\############||||#||###||\/#| |||\#\/#||#\#\#||#\//\##\/########\#||# |#||||#/\########/--\/\||||#||###||#\#| |\/\|\##||##|\#\/###||#/\#/\/\|\###|'|# |#||\/#|||\|-----' |||||||#\/###|||\#|,/\||||##||##||##|\##||/\/\||||||###| |# |#||###||||| |||||||##|\##||||/\/||||||##||##||##||##||||||||||||###| |# |#||###||||| |||||||##||##||||\/#||||||##||##||##||##||||\/||||||###| |# |#\/###||\/' |||||||#/\/##\/\/\\#\/\/||##||##||#/\/##\/\/#\\/\/||#/\| |# |##|\##\/#| |||||||#\/####|\\/##\\##\/##\/##||#\/#/\#\##\###\#\/#\/\.|# |##|||\#|\| |\/|||||\#\###\/#######\###|\###|||\#\\/\#\############\||# |##\/\/#\/| `\#\/||\/#|\\########/\####\/###||\/#|\\/####|\#########||# |###/\\\/'' `'--'`-'-'`-'\\/-\\\\/\\---\/--'`-'-'`-\\#\\'|\/\##/\/\\/# |###\/##\_________________________/#\_/###\/#\__/\__________/####\/#\/##\/\/### `-\##########################################################################/- `--------------------------------------------------------------------------'
VT100 animation
the VT100 Animation files are meant to be thrown up raw at a vt100-compatible terminal. The files themselves are simply text with cursor movement instructions, deleting and erasing the characters necessary to appear animated. Usually, they represent a long hand-crafted process done by a single person to tell a story. Some of these files may date back to the 1960's and 1970's.
you can find these all vt100 files in this link : artscene.textfiles.com/vt100/
execute VT file in terminal:
1. Copy the VT file link.
2. then execute it with curl command. Change the speed in the pv command, here is an example :
curl -s http://artscene.textfiles.com/vt100/cow.vt | pv -q -L 4400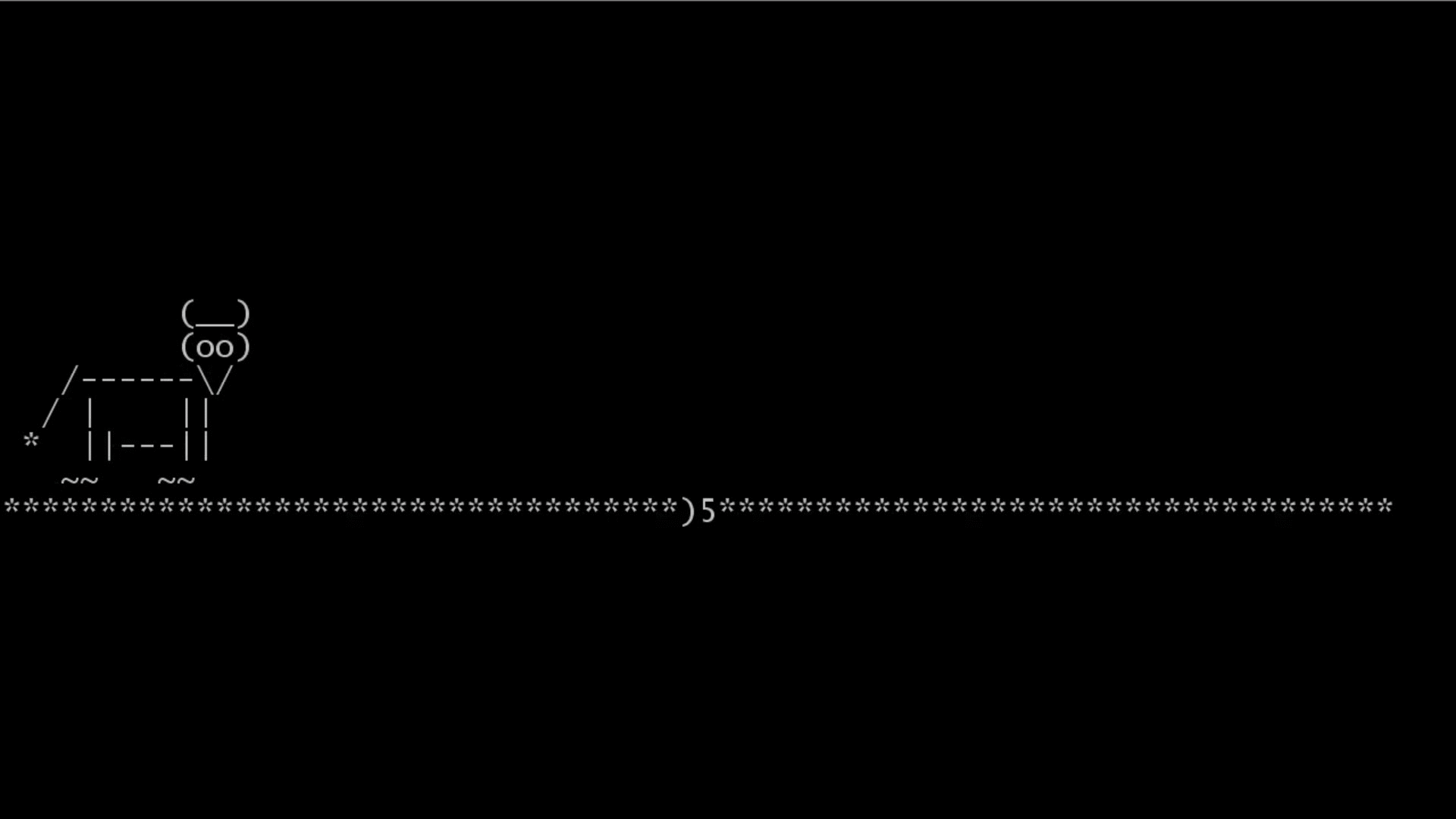
Star Wars
The ASCII version of the Star Wars IV movie by Simon Jansen uses the same idea of the ASCII animation process, this ASCII movie is about 18 min 30 sec.
you can watch the full movie at this link : asciimation.co.nz
WWW.AS
SL Linux command
SL is a joke software or classic UNIX game. It is a steam locomotive that runs across your terminal if you type “sl” (Steam Locomotive) instead of “ls” by mistake. SL is a highly advanced animation program for curing your bad habit of mistyping. In other words, you see a Steam locomotive in ASCII art.
install it by this command :
sudo apt-get install slthen just type sl.
sl(@@@)
( )
(@@@@)
( )
==== _________ ___________
_D _| |_______/ STASCII \__I_I____===__|_________|
|(_)--- | H\_________/ | | =|___ ___| _________________
/ | | H | | | | ||_| |_|| _| \_____A
| | | H |__--------------------| [___] | =| |
| ________|___H__/__|_____/[][]~\_______| | -| |
|/ | |-----------I_____I [][] [] D |=======|____|________________________|_
__/ =| o |=-~~\ /~~\ /~~\ /~~\ ____Y___________|__|__________________________|_
|/-=|___|= O=====O=====O=====O|_____/~\___/ |_D__D__D_| |_D__D__D_|
\_/ \__/ \__/ \__/ \__/ \_/ \_/ \_/ \_/ \_/
batch animation
if you using Microsoft Windows, then you can also create an ASCII animation using batch files (.bat) and execute it in the CMD. But in this case, you can't use some symbols such as " | " and " > " because batch files consist of a series of commands to be executed by the command-line, and some characters have special meanings in echo command.
here is an example of a globe animation created with batch on Github : Earth ASCII Animation.bat
.-/+osyysooooo+/:. `:osshohhhs++/. :dddddho:` :os@@:` `. :o/`s@h-s@@@@@s: -sNNdyy@/ -: @y@@@@@@@@@@@@Ny: /dN@d++ `` :/+@@yy:h@@@dsNN@@@+` /@@d+` o@@d: .+++dys@d@@dN+ .d@h- -o@@@@@@@d:--::://y@@N@@- /@d. :oN@@@@@@@@@@@@@@@@@Nh@@@N@o oy`: y@@@@@@@@@@@@@@@@@@@@yhy@@@@s +d. o :o@@@@@@@@@@@@@@@@@@@@@@@/h@@@ys -@- ` y@@@@@@@@@@@@@@@@@@@@@@@@N@/N@/d: h+/ ```` odN@@@@@@@@@@@@@@@@@@@@@@@@@s-.:@ .N `y@@@N--. +h@@@@@@@hddN@@@@@@@@@@@@@@@+`d: /h `y@@@@@@h:` /yyyyy/ `ohN@@@@@@@@@@@@d/`so oy /@@@@@@@@@+//- h@@@@@@@@@@@@/``oy +h :@@@@@@@@@@@@h+++` d@@@@@@@@@@@/```ss .N `@@@@@@@@@@@@@@@@: /s@@@@@@@@@@````d: d/ -N@@@@@@@@@@@@d/. /@@@@@@@@@o```:@ -@` `s@@@@@@@@@@@@ :h@@@@@@@@@N:@o.d/ oy .N@@@@@@@@@@. `-N@@@@@@@+.yh.sy sy .N@@@@@@@+` o@@@@@@ds`:/`oy +h` -N@@@@@No `sN@@@ds````.ys -h/ .d@@@@s` `/N++/-````/d: +h: +N@y` ```..``````:yo` `+y/:@y` ``````````/yo` :sss+: `````````:sy/ `/ss+- ```````-+ss/` ./oooys:. ``::///++oosso/. `-:+ooooshhhhyso+/-`